A linked list is a list of elements linked together. Each element is represented by a node which consists of two components - information about that node, i.e., the data stored in the node, and a pointer to the next node in the list. We need to maintain a node named 'start' (it can be arbitrarily named) to mark the beginning of the list. The last element points to NULL.
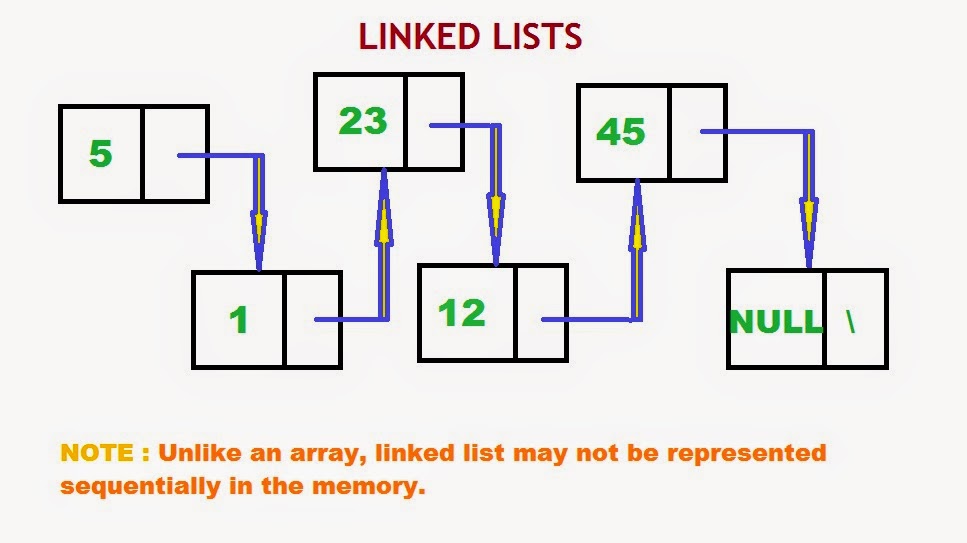 |
Linked Lists |
Insertion into linked list
For inserting a new element in the linked list, we need to follow these steps :
2. Set the data of the node as the value to be inserted
3. Set the next pointer of the node to NULL.
4. a.If start is NULL, set root as the new node
b. If start is not NULL, set the last element's next pointer as the new node
Here's the code for insertion :
Traversal in a linked list
For traversal in a linked list, we need the start node, which tells us the start of the list. We take a temporary pointer, initialise it with the start pointer, and move the temporary pointer to the next node till it reaches NULL.
Here's the code for traversal :
Here's a complete program including the above functions :